Action bar
This week I will be creating an action bar for my game that will be select-able by the player so that the wizard character can shoot up to 3 different spells, as currently you can only shoot a fireball in my game and I want variation to the spells I can cast.
So firstly I will have to bring in some extra sprites into my game for the different spells I want to use, including the action bar. I created a new folder to organize my new sprites under 'UI' as they will be used for the user interface for the player to click on:
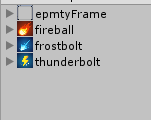
As you can see there are 3 different spell sprites and also an empty frame sprite that each of the spells will be contained in.
Next in the Unity editor I added an image which will be serving as the container for the action bar, so I named this image 'action bar':
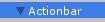
I placed the action bar image at the bottom of the players view, stretched from left to right.
Next within my action bar image I created 'buttons' for each of my spells and I assigned all 3 spell sprites to them:
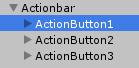
I also added the frame sprite to each of them:
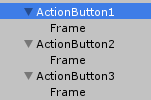
I then played around with the settings in Unity so that my spells are aligned in the middle of the players screen, as if left alone the spells are automatically placed to the left hand side of the action bar image:
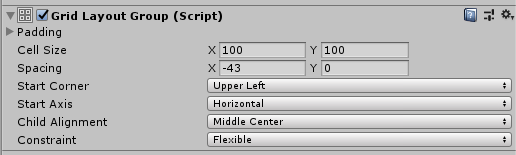
After playing around with the alignment settings this is how my sprites look on the user interface:
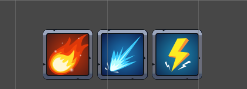
Now I need these spells to have a button component, so to do this I selected all the of spells in the Unity editor, selected add component on the right hand side and selected 'button' which will perform as an 'on click' function.
Before I can create the scripts for the spell select functionality, I need to sort out the prefabs for the other 2 spells. So to do this I simply duplicated my already created fire ball prefab and assigned the appropriate spell sprite to them:
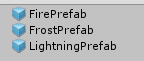
Script
Now that the assets are in the game it's time to create some functionality scripts to make it so the spells are select-able on the action bar.
I need to use a specific spell when casting, so first I created an index:


We are going to be using the function that instantiates spells to call from the spell index, because before it just instantiated one spell (fire ball) but now there will be an index of different spells to cast:

After this, on the button component I created earlier, I added the player script to that button and selected the cast spell function for the button functionality. I did this for each of the action buttons:

After this my spell selecting functionality should work, however it doesn't. The issue I ran into is that when I select the spell then select the enemy to fire at, it de-selects the spell, because of how it works is that once you select something that isn't the spell after selecting it, it will de-select. So I needed to fix this.
So to fix this we need to change something in the game manager, I need to add an extra condition to make sure I dont de target the enemy:

The line of code highlighted is the part I just added, it checks if the mouse is hovering over a UI element on the current event system, which means if the mouse is hovering over lets say the fireball spell, the code wont execute. The code will only execute if the mouse is not over a UI element, the only time it should de target the enemy is if I click somewhere outside the UI elements, like any other part of the map.
Now in game, I can use the mouse button to throw spells at the enemy. However, I would like to add extra control functionality, like 1, 2 and 3 on the keyboard, because using the mouse button slows down the pace of the game and key bindings I find is a lot more easier and fun to use in a gaming environment.
So I will be creating a new script, it will be called UIManager:
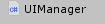
The UI manager will have a reference to all the buttons so that I can set key binds to the buttons.
First to create a reference to the action buttons:
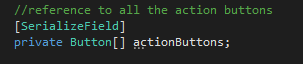
The I created 3 different key codes from 1 to 3, which will be used for executing the action buttons via key press:

Then I created the 3 different keybindings for 1, 2 and 3 on the keyboard:
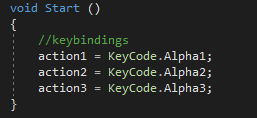
Now for creating the function which will allow use to execute the keybindings from the key bindings index:
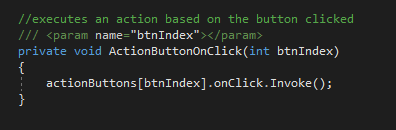
After that, I created the getkeydown and the onClick functions for each of the spells on the action bar:
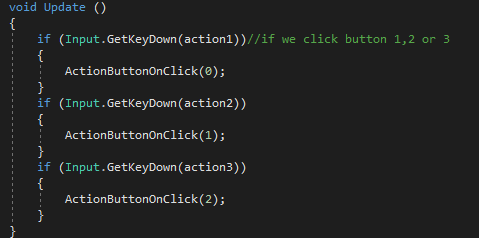
After that I am finished, my spells now work by either clicking on them with the left mouse button, or the 1, 2 and 3 keys.
Here is what it looks like in game currently:
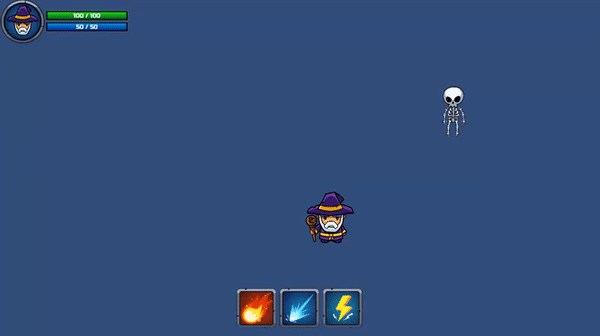
As you can see my action bar is looking very nice and the functionality works great. After clicking on each of the different spells, different prefabs fire out of the wizards staff, i'd also like to point out that the keybindings do also work but it's impossible to show that on video/gif. In the future I would like to make it so the spells damage the enemy and apply different effects based on the different spells I use, for example using a fire ball may cause the enemy to have a burning effect applied, and using forst may have some sort of slowing effect, but that will be added way further in development, for now I am happy with how things are.
This week I will be creating an action bar for my game that will be select-able by the player so that the wizard character can shoot up to 3 different spells, as currently you can only shoot a fireball in my game and I want variation to the spells I can cast.
So firstly I will have to bring in some extra sprites into my game for the different spells I want to use, including the action bar. I created a new folder to organize my new sprites under 'UI' as they will be used for the user interface for the player to click on:
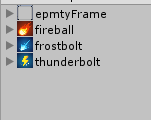
As you can see there are 3 different spell sprites and also an empty frame sprite that each of the spells will be contained in.
Next in the Unity editor I added an image which will be serving as the container for the action bar, so I named this image 'action bar':
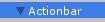
I placed the action bar image at the bottom of the players view, stretched from left to right.
Next within my action bar image I created 'buttons' for each of my spells and I assigned all 3 spell sprites to them:
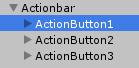
I also added the frame sprite to each of them:
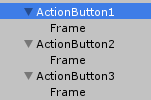
I then played around with the settings in Unity so that my spells are aligned in the middle of the players screen, as if left alone the spells are automatically placed to the left hand side of the action bar image:
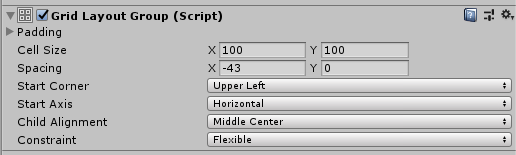
After playing around with the alignment settings this is how my sprites look on the user interface:
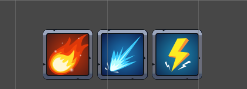
Now I need these spells to have a button component, so to do this I selected all the of spells in the Unity editor, selected add component on the right hand side and selected 'button' which will perform as an 'on click' function.
Before I can create the scripts for the spell select functionality, I need to sort out the prefabs for the other 2 spells. So to do this I simply duplicated my already created fire ball prefab and assigned the appropriate spell sprite to them:
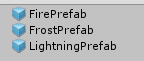
Script
Now that the assets are in the game it's time to create some functionality scripts to make it so the spells are select-able on the action bar.
I need to use a specific spell when casting, so first I created an index:


We are going to be using the function that instantiates spells to call from the spell index, because before it just instantiated one spell (fire ball) but now there will be an index of different spells to cast:

After this, on the button component I created earlier, I added the player script to that button and selected the cast spell function for the button functionality. I did this for each of the action buttons:

After this my spell selecting functionality should work, however it doesn't. The issue I ran into is that when I select the spell then select the enemy to fire at, it de-selects the spell, because of how it works is that once you select something that isn't the spell after selecting it, it will de-select. So I needed to fix this.
So to fix this we need to change something in the game manager, I need to add an extra condition to make sure I dont de target the enemy:

The line of code highlighted is the part I just added, it checks if the mouse is hovering over a UI element on the current event system, which means if the mouse is hovering over lets say the fireball spell, the code wont execute. The code will only execute if the mouse is not over a UI element, the only time it should de target the enemy is if I click somewhere outside the UI elements, like any other part of the map.
Now in game, I can use the mouse button to throw spells at the enemy. However, I would like to add extra control functionality, like 1, 2 and 3 on the keyboard, because using the mouse button slows down the pace of the game and key bindings I find is a lot more easier and fun to use in a gaming environment.
So I will be creating a new script, it will be called UIManager:
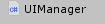
The UI manager will have a reference to all the buttons so that I can set key binds to the buttons.
First to create a reference to the action buttons:
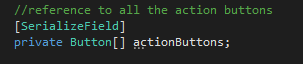
The I created 3 different key codes from 1 to 3, which will be used for executing the action buttons via key press:

Then I created the 3 different keybindings for 1, 2 and 3 on the keyboard:
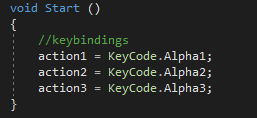
Now for creating the function which will allow use to execute the keybindings from the key bindings index:
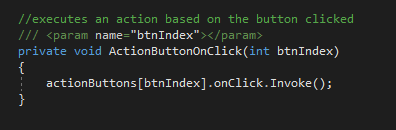
After that, I created the getkeydown and the onClick functions for each of the spells on the action bar:
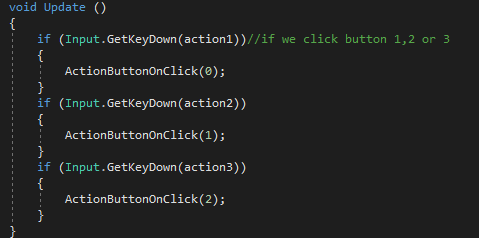
After that I am finished, my spells now work by either clicking on them with the left mouse button, or the 1, 2 and 3 keys.
Here is what it looks like in game currently:
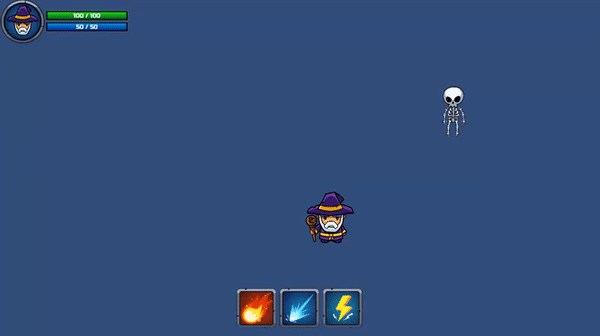
As you can see my action bar is looking very nice and the functionality works great. After clicking on each of the different spells, different prefabs fire out of the wizards staff, i'd also like to point out that the keybindings do also work but it's impossible to show that on video/gif. In the future I would like to make it so the spells damage the enemy and apply different effects based on the different spells I use, for example using a fire ball may cause the enemy to have a burning effect applied, and using forst may have some sort of slowing effect, but that will be added way further in development, for now I am happy with how things are.
Comments
Post a Comment