Now I am going to make the
enemy follow the player around when the player gets too close to the enemy,
meaning the enemy won’t always follow the player from the get go, but once in
range the enemy will notice the player and start to follow him. For now it will
be a simple path finding function that will only follow the player, but I later
plan on adding a more advanced version which allows the enemy to detect obstacles
in its way so it can move around them.
So in order to make the
enemy follow the player it will first need to be able to see the player, to do
this we need to add a ‘range’ to the enemy, which is done by creating a 3D
object within the unity editor (not the code):

The range is going to decide
when the enemy is able to see the player, so I will need to add a circle
collider to the 3D object:
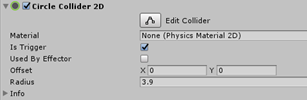
This circle collider is what
it says on the tin, it’s a circle around the enemy which is attached to him so that
it follows him at all times, once the player steps within the range of this
circle, the player is then visible to the enemy. I can make the circle size to
whatever I want, which will allow me to increase the sight range at will which
will be useful for other enemies later on, for example making a bigger circle
for ‘harder’ enemies which will see you at a further distance.
Script:
So in order for the range to
see the player we will need to do some scripting, so first let’s make a script
called ‘Range’:

And then simply add the
script to the range object in Unity:

In the range script we need
to make sure once the range is triggered that the enemy will proceed to follow
the player, to do that we need to give the enemy a target, so in the enemy
script we need to call for a target function like so:

So now we can set the
target, we can do this in the range script:

So here if the player
collides with the range we need to set it as a target
Our enemy has the range as a
child object which means we need to access the enemy script within the parent
and we need to set the target on that enemy script
So to do this we create this
code here, which makes sure the enemy script is referenced within the range
script:
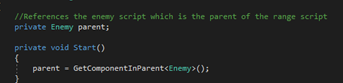
So here I created code which
once the player collides with the range, the player becomes the targets
transform:

Now that the enemy will be
able to target the player, we need to add some movement functionality, so to do
this we need to go back to the enemy script and create a function called FollowTarget:

After that we need to change
the enemy’s position based off the player’s position, so I created code that
moves the enemy towards the players position, and it will continue to follow to
the player’s position so long as the player keeps moving, if the player does
not move, the enemy will move and stop at the players position entirely:

However, the enemy will not
follow the player just yet because the function hasn’t been updated within the
enemy script so to do this we add this:
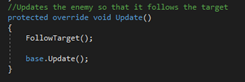
Now that has been done, the
enemy should update to the FollowTarget functionality and follow the player
However if I start the game
in its current state the enemy won’t follow the player just yet, and that is
because we haven’t set a speed for the enemy within the Unity editor
Here is the value I entered
in the editor; you can enter whichever value you like, the higher the value the
faster the enemy will follow you. Currently 0.8 is a low speed and I may change
it in the future:

In this current state, the
enemy will infinitely follow the player no matter where the player runs to, so
after the player collides with the range the enemy will follow the player
indefinitely, and I don’t want that. I want to change it so if the player moves
out of the enemies range, the enemy will give up on follow the player until he
re-enters the range again.
So to make sure we can out
run the enemy we need to go back to the range script and add this line of code:
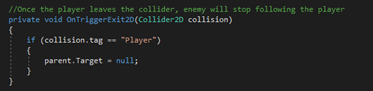
This is exactly the same as
the code which allows the player to become a target with a few adjustments, so
if the player leaves the trigger(exit) then the target becomes null, and with a
null target the enemy has no one to follow, and will remain in place.
Now all the script has been
created, the enemy will follow my player character around in game!
Comments
Post a Comment