Target system
This week I will be setting up a target functionality for the game, what this means is instead of my spells being auto directed to the nearest enemy you will have to instead select the target you want to attack with left click and then proceed to attack it. The current system in place is more of a debugging combat system and I intended for the game to have target select mechanics before hand, which I will be adding this week.
Script
First off I started by removing the current targeting system altogether, as we won't be using it any longer. Then, I replaced the previous private target property with a public transform:

Adding this property with 'target' initially gave me some errors basically saying that 'target' doesn't exist and won't function, so I changed target to 'MyTarget' which fixes this error as I have used MyTarget before in my LineOfSight function which I added last week.
The reason I created this property instead of the other one is because I want to set the target from else where, i'll be creating a game manager that will handle the whole targeting system and will tell the player which object they last clicked on was.
Next I created a new script called 'GameManager'

Firstly the game manager needs a reference to the player:
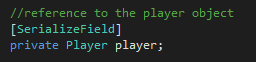
As well as the script, I also created an object within unity editor also called game manager:
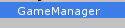
And I added both the new game manager script and the player script to this new object:

Next I needed to create a new function that will allow clicking on a target within the game manager:

First the game needs to check if I click mouse button 1 (left click), and to do that I will create an if statement

This line checks if we are clicking the left mouse mouse, the 0 value is the left mouse button, so a value of '1' would be the right mouse button, so this can be changed to either values depending on if I want my targeting to be via left or right click, but traditionally in RPG games left click preforms most actions, including targeting, so I will keep it at 0.
Next we need to create a RayCast, this Ray will be cast from the mouse position into the games world, and it will store the ray cast inside 'hit'

What this code pretty much means is that the mouse position will be translated into a world position within the game world. The value at the end is the layer value which I added also, so the ray cast will only hit objects available on that specific layer.
I added a layer mask, which pretty much makes it so not everything in the world will be target able as I only want certain things able to be targeted, for example I want to target enemies but not things like trees, which I will be adding later on.
So to add a layer I selected target within the Unity editor, clicked on layers in the side panel and added a new layer which I dubbed 'clickable' which will be everything the player can click. The layer before that is 'block' which will be everything else in the world, this layer has a different value to clickable, half the value to be precise.
Now for the if statement:

So if the ray cast is null, we didn't hit anything, if it's NOT null then it will set the players target which we can cast spells at

So now we will need to un target if successful, so to do this by setting myTarget to null (nothing)
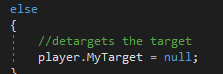
So for me to be able to select the enemy as a target I will need to create a box collider
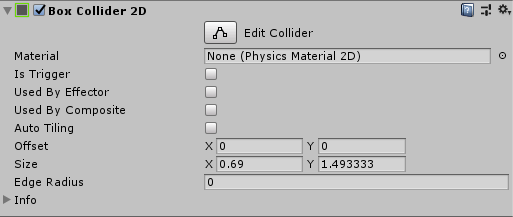
And that's everything! Now, for me to be able to attack the enemy I will first need to click on him to select him as a target, once I do that I can attack the target. Also, if I click off the enemy (as shown below, although its hard to tell) it will deselect it as a target so I am then unable to attack anything. I will need to add GUI in the future to indicate this system entirely, for example once I select the target I want it to show up at the top of the screen with the enemies icon, also its health, which I haven't added yet but I will at some point in development.
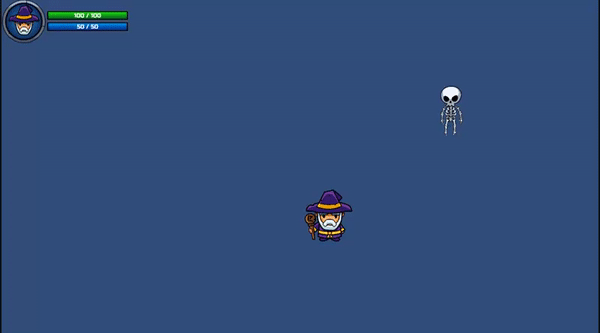
This week I will be setting up a target functionality for the game, what this means is instead of my spells being auto directed to the nearest enemy you will have to instead select the target you want to attack with left click and then proceed to attack it. The current system in place is more of a debugging combat system and I intended for the game to have target select mechanics before hand, which I will be adding this week.
Script
First off I started by removing the current targeting system altogether, as we won't be using it any longer. Then, I replaced the previous private target property with a public transform:

Adding this property with 'target' initially gave me some errors basically saying that 'target' doesn't exist and won't function, so I changed target to 'MyTarget' which fixes this error as I have used MyTarget before in my LineOfSight function which I added last week.
The reason I created this property instead of the other one is because I want to set the target from else where, i'll be creating a game manager that will handle the whole targeting system and will tell the player which object they last clicked on was.
Next I created a new script called 'GameManager'

Firstly the game manager needs a reference to the player:
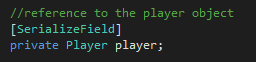
As well as the script, I also created an object within unity editor also called game manager:
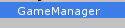
And I added both the new game manager script and the player script to this new object:

Next I needed to create a new function that will allow clicking on a target within the game manager:

First the game needs to check if I click mouse button 1 (left click), and to do that I will create an if statement

This line checks if we are clicking the left mouse mouse, the 0 value is the left mouse button, so a value of '1' would be the right mouse button, so this can be changed to either values depending on if I want my targeting to be via left or right click, but traditionally in RPG games left click preforms most actions, including targeting, so I will keep it at 0.
Next we need to create a RayCast, this Ray will be cast from the mouse position into the games world, and it will store the ray cast inside 'hit'

What this code pretty much means is that the mouse position will be translated into a world position within the game world. The value at the end is the layer value which I added also, so the ray cast will only hit objects available on that specific layer.
I added a layer mask, which pretty much makes it so not everything in the world will be target able as I only want certain things able to be targeted, for example I want to target enemies but not things like trees, which I will be adding later on.
So to add a layer I selected target within the Unity editor, clicked on layers in the side panel and added a new layer which I dubbed 'clickable' which will be everything the player can click. The layer before that is 'block' which will be everything else in the world, this layer has a different value to clickable, half the value to be precise.
Now for the if statement:

So if the ray cast is null, we didn't hit anything, if it's NOT null then it will set the players target which we can cast spells at

So now we will need to un target if successful, so to do this by setting myTarget to null (nothing)
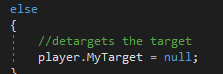
So for me to be able to select the enemy as a target I will need to create a box collider
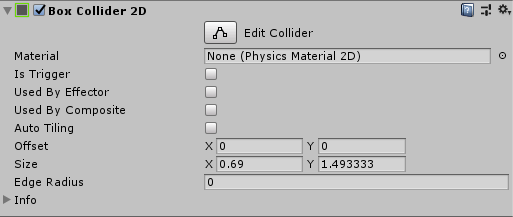
And that's everything! Now, for me to be able to attack the enemy I will first need to click on him to select him as a target, once I do that I can attack the target. Also, if I click off the enemy (as shown below, although its hard to tell) it will deselect it as a target so I am then unable to attack anything. I will need to add GUI in the future to indicate this system entirely, for example once I select the target I want it to show up at the top of the screen with the enemies icon, also its health, which I haven't added yet but I will at some point in development.
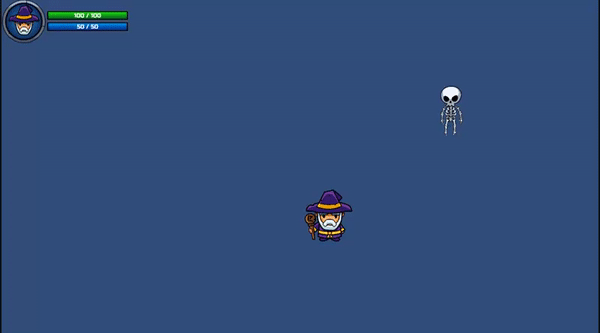
Comments
Post a Comment