Enemy health bar overview
In this week of development I will be creating a unit frame and health bar for the enemies in my game, meaning every time I target an enemy in my game it will show a unit frame at the top of my screen and also a health bar above my enemy. A unit frame is basically a UI element that showcases what type of enemy you are targeting, so in my case if I target the enemy skeleton then UI will pop up on the screen with a picture of the skeleton, and a health bar will be similar to what my character has already except for the enemy.
Note
I'd also like to point out I have added a cast time for my game but didn't document the process of this, I may add all the code and stuff at the end of the documentation and explain briefly what I did, but to sum it up every time my character casts a spell now there is a cast time and UI for each different spell.
Creating the health bar
For creating the enemy health bar I will be using the same method that I used for creating the players health bar, except with the extra things like mana bar. So firstly in the unity editor I added a object on the target (enemy) called HealthCanvas:
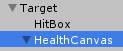
The health canvas will have the health bar underneath it. To make sure the canvas follows with the enemy when it moves around I needed to change one of the options in the unity editor to this:

After that I added the background under the health canvas, this will be the background full the health bar (black):
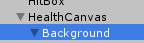
It was originally quite large in the world so I scaled it down to a reasonable size.
Next I added a child object called fill which will act as the visualization of the health bar going down and I made it a red color to indicate health:
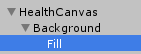
Script
Now for the scripts, so to start off I will be creating 2 new scripts, one called NPC which I will be using later and enemy.
NPC will be inhertting functionalities from the character script, so that for the future it will make things easier when I start to add things like quests:

And then the enemy script will be inheriting from the NPC script:

Next to create a private canvas group called healthGroup as I would like to show the health bar when we click on the enemy:

Then in game manager I created a private NPC which will be the code for the current target:

So when we hit something it needs to check if the current target isn't null:

Because if we have a target already we need to de select the target we already have.
Now to add a premature script which I will add functionality later for, but so I can call for it I added this in the NPC script:
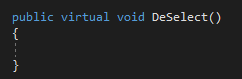
Then in the game manager I added a deselect function, so I hit a target and i have a target already it will de select the target:

Then this code gets the NPC script functionality and selects a new target:

Then I need to return a transform from my current target, so to do this I added a new function called select, which I created in the NPC script. The select function needs to return the transform which is actually the hitbox for the NPC. So in the character script I created a transform called hitbox:
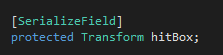
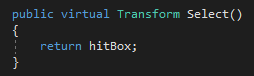

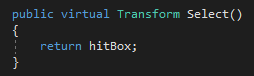
Also if my target isn't null (anywhere in the game world that isn't a clickable target) and I have a current target, then my current target deselcts:

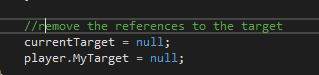
Now for the enemy script, I created a health group and set the alpha number to 1 making it so we can see the health bar above the enemy:
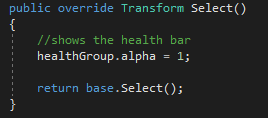
Now the script is done, I went back into Unity and added the enemy script to the target so it takes its functionalities:

So now when I load up my game I should be able to view the enemy health bar after clicking on the enemy:
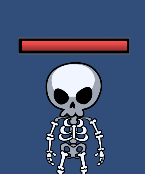
The only problem now is the health bar is not removed from the screen once we deselect the enemy, so to fix this I created an override called de select which will change the alpha number to 0 (not visible):
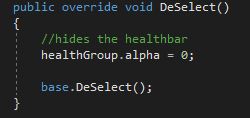
So now I can select the target to display the health bar and I can also click off the target to deselect it.
In the future I plan on making the enemy able to die and reduce the health bar.
In this week of development I will be creating a unit frame and health bar for the enemies in my game, meaning every time I target an enemy in my game it will show a unit frame at the top of my screen and also a health bar above my enemy. A unit frame is basically a UI element that showcases what type of enemy you are targeting, so in my case if I target the enemy skeleton then UI will pop up on the screen with a picture of the skeleton, and a health bar will be similar to what my character has already except for the enemy.
Note
I'd also like to point out I have added a cast time for my game but didn't document the process of this, I may add all the code and stuff at the end of the documentation and explain briefly what I did, but to sum it up every time my character casts a spell now there is a cast time and UI for each different spell.
Creating the health bar
For creating the enemy health bar I will be using the same method that I used for creating the players health bar, except with the extra things like mana bar. So firstly in the unity editor I added a object on the target (enemy) called HealthCanvas:
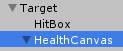
The health canvas will have the health bar underneath it. To make sure the canvas follows with the enemy when it moves around I needed to change one of the options in the unity editor to this:

After that I added the background under the health canvas, this will be the background full the health bar (black):
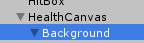
It was originally quite large in the world so I scaled it down to a reasonable size.
Next I added a child object called fill which will act as the visualization of the health bar going down and I made it a red color to indicate health:
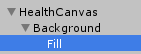
Script
Now for the scripts, so to start off I will be creating 2 new scripts, one called NPC which I will be using later and enemy.
NPC will be inhertting functionalities from the character script, so that for the future it will make things easier when I start to add things like quests:

And then the enemy script will be inheriting from the NPC script:

Next to create a private canvas group called healthGroup as I would like to show the health bar when we click on the enemy:

Then in game manager I created a private NPC which will be the code for the current target:

So when we hit something it needs to check if the current target isn't null:

Because if we have a target already we need to de select the target we already have.
Now to add a premature script which I will add functionality later for, but so I can call for it I added this in the NPC script:
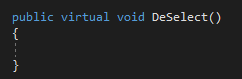
Then in the game manager I added a deselect function, so I hit a target and i have a target already it will de select the target:

Then this code gets the NPC script functionality and selects a new target:

Then I need to return a transform from my current target, so to do this I added a new function called select, which I created in the NPC script. The select function needs to return the transform which is actually the hitbox for the NPC. So in the character script I created a transform called hitbox:
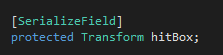
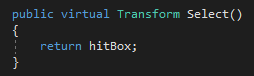

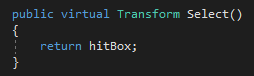
Also if my target isn't null (anywhere in the game world that isn't a clickable target) and I have a current target, then my current target deselcts:

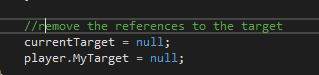
Now for the enemy script, I created a health group and set the alpha number to 1 making it so we can see the health bar above the enemy:
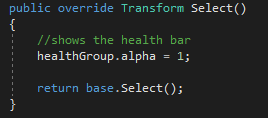
Now the script is done, I went back into Unity and added the enemy script to the target so it takes its functionalities:

So now when I load up my game I should be able to view the enemy health bar after clicking on the enemy:
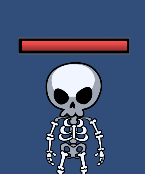
The only problem now is the health bar is not removed from the screen once we deselect the enemy, so to fix this I created an override called de select which will change the alpha number to 0 (not visible):
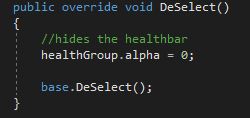
So now I can select the target to display the health bar and I can also click off the target to deselect it.
In the future I plan on making the enemy able to die and reduce the health bar.
Comments
Post a Comment