For this week I will make it so the playable character is able to kill the enemies instead of just firing at a useless target dummy. But before that there is a little bug that I have to fix where when the character attacks the enemy then quickly de targets the enemy, I get a null reference exception because we can't reach whatever is in line of sight and the spell casting carries on.
Bug fix code
Firstly I navigated to the in line of site code line which is what is creating the error, and to fix it we I need to add some code which checks if we have a target before we un target:
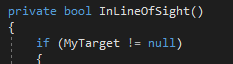
So if we don't have a target, then cancel the spell cast, if we do then carry on casting:
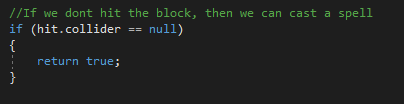
Enemy kill code
Now that little bug is fixed I can now add some code which will make it able to damage the enemy skeleton, because the nature of an RPG game is all about damage, whether its the character damaging enemies or the character itself taking damage. So to start off I created a virtual void named TakeDamage:

So next I need to make it reduce health, however I don't currently have health for the enemy so I will have to create that too. First off I added a 'stat' script to the enemies health canvas:

After that in the character script I added the stat in there too:
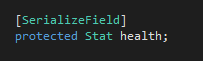
After that I added a line of code which equals to taking damage:
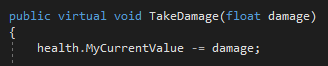
Next we need the spell to actually deal damage, so to do this in the spell script I added a line of code so the parent (enemy) takes damage from the spell:

But it won't deal damage just yet, so firstly I needed to create an integer called damage:

This line of code will transform the targets health upon taking damage:
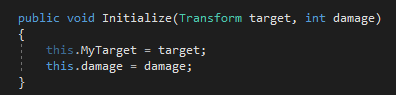
Currently the spell hits the target and disappears on impact, there will be a delay for the enemy to disappear because of the damage animation, so to make sure the spell doesn't bug out on the screen I set the speed to 0 here:

And to make the damage reducing factor work properly I had to move this line of code from the player script into the character script, as we are working with the enemies health and not the player itself:

Also in the Unity editor I had to change the health bar from simple to 'filled' so that the health bar horizontally goes down, as with simple turned on it doesn't move at all even though we are dealing damage:

So now that we are able to damage the enemy, I need to make it so we are able to kill him once his health hits zero. For this I will be using assets I have attained of a death animation for the enemy, as seen here:
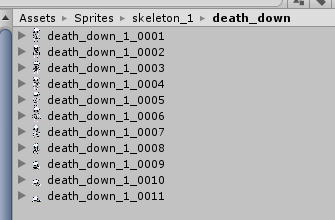
For the animation to take effect I will be working with the Unity Animator window again, idle is the default state of the enemy for when he is alive, die_down is the animation played when his health hits zero. So I need to create a trigger for the die animation, so to do this in the character script I added a line of code which means if the health reaches zero set off the die animation trigger:
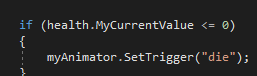
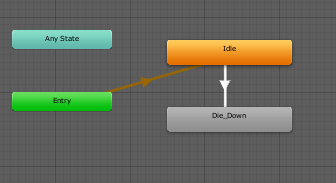
So now we are able to attack and kill the enemy with a death animation, however I am still able to attack the enemy once he is dead and I would like to change that, so to do this I need to create a behavior within the animator for our death animation, in the death behavior script I added a line of code which will destroy my target so we can't attack it anymore :

So now that we can't attack the target once it is dead I would like the corpse of the enemy to disappear from the game, so to do this I added a timer for the corpse to stay in the game for which is 5 seconds, once 5 seconds are up then destroy the game object (enemy) from the scene:
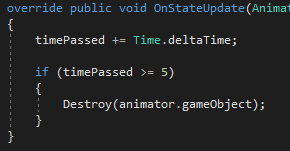
Here is what damaging my enemy and killing him looks like in game:


Bug fix code
Firstly I navigated to the in line of site code line which is what is creating the error, and to fix it we I need to add some code which checks if we have a target before we un target:
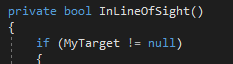
So if we don't have a target, then cancel the spell cast, if we do then carry on casting:
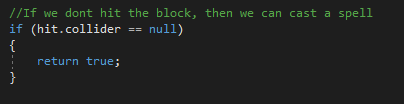
Enemy kill code
Now that little bug is fixed I can now add some code which will make it able to damage the enemy skeleton, because the nature of an RPG game is all about damage, whether its the character damaging enemies or the character itself taking damage. So to start off I created a virtual void named TakeDamage:

So next I need to make it reduce health, however I don't currently have health for the enemy so I will have to create that too. First off I added a 'stat' script to the enemies health canvas:

After that in the character script I added the stat in there too:
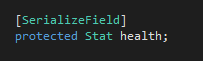
After that I added a line of code which equals to taking damage:
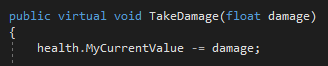
Next we need the spell to actually deal damage, so to do this in the spell script I added a line of code so the parent (enemy) takes damage from the spell:

But it won't deal damage just yet, so firstly I needed to create an integer called damage:

This line of code will transform the targets health upon taking damage:
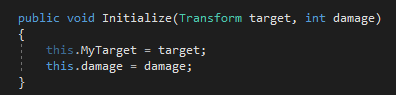
Currently the spell hits the target and disappears on impact, there will be a delay for the enemy to disappear because of the damage animation, so to make sure the spell doesn't bug out on the screen I set the speed to 0 here:

And to make the damage reducing factor work properly I had to move this line of code from the player script into the character script, as we are working with the enemies health and not the player itself:

Also in the Unity editor I had to change the health bar from simple to 'filled' so that the health bar horizontally goes down, as with simple turned on it doesn't move at all even though we are dealing damage:

So now that we are able to damage the enemy, I need to make it so we are able to kill him once his health hits zero. For this I will be using assets I have attained of a death animation for the enemy, as seen here:
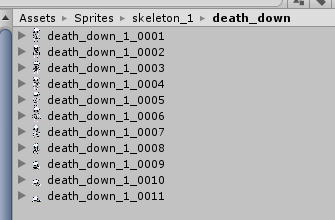
For the animation to take effect I will be working with the Unity Animator window again, idle is the default state of the enemy for when he is alive, die_down is the animation played when his health hits zero. So I need to create a trigger for the die animation, so to do this in the character script I added a line of code which means if the health reaches zero set off the die animation trigger:
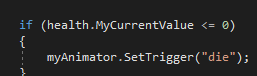
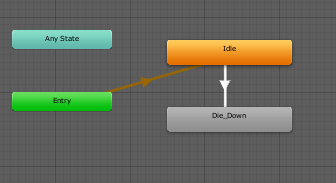
So now we are able to attack and kill the enemy with a death animation, however I am still able to attack the enemy once he is dead and I would like to change that, so to do this I need to create a behavior within the animator for our death animation, in the death behavior script I added a line of code which will destroy my target so we can't attack it anymore :

So now that we can't attack the target once it is dead I would like the corpse of the enemy to disappear from the game, so to do this I added a timer for the corpse to stay in the game for which is 5 seconds, once 5 seconds are up then destroy the game object (enemy) from the scene:
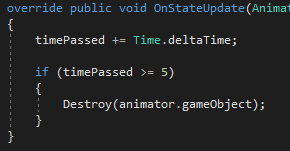
Here is what damaging my enemy and killing him looks like in game:


Comments
Post a Comment