This week I will be adding some GUI to my game so that the enemy has a sort of unit frame when he is selected that will pop up at the top of the screen to indicate what target you currently have selected in the game. It will work similarly to the players GUI in that it will display a picture icon and the targets health.
Enemy GUI frame
The easiest way to create this would be to duplicate the already existing frame and rename and tweak it a little so that it corresponds as a target frame instead:

The target icon will be displayed to the right of the health bar in contrary to the player which is to the left, just to mix things up a bit. I also changed the portrait from the players face to an enemy skeleton, which was a simple change done within the editor. Next I removed the mana bar because the enemy won't be using magic, I then changed the color of the bar to red from green which is more fitting color for an enemy, green is good red is bad.
Script
First things first I needed to create a game object for the GUI which was done in the UIManager script.

Then I created a new function called showTargetFrame which will show the enemies target frame once clicking on the target. This function works for not only enemy targets but friendly targets such as quest givers or vendors:

This line of code sets the frame to show:

Next we need to call this function, this can be done in the gameManager script inside the click target function. First thing I did was create a private static instance in UIManager called UIManager:

Next I made it so if instance is null then we have to set it. This makes sure when I access the UIManager through myInstance we check if the instance is set if not we set it then return it:
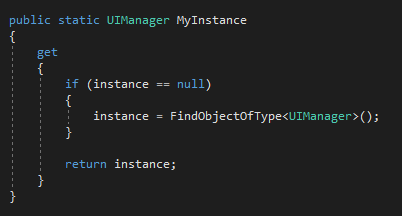
Then within gameManager I put this line of code which allows the gameManager script with the click function to access my UIManager script and the showTarget functionality:

After this the target GUI will display at the top of the screen when selecting it. Now I need to make it so when we unselect the target the GUI goes away. Pretty simple way of doing that:
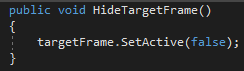
Currently the health doesn't update, so I will have to initialize the health on the target frame. To do this I will create a health stat in the character script:
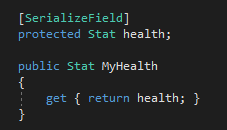
Then within the show target frame function I create this line of code which initializes the health for the selected target which sets its max health value and its current value:

Then to allow this functionality to work from the get go I added this line of code within the void start within the ui manager script:

Now when I go into the game and select the target the correct information about the target will be displayed at the top. However at this stage there is no functionality for updating the health once the player damages it, so I will need to add that.
So to do this I will be creating a delegate, so in the NPC script I created a delegate called HealthChanged, and the function that it will be changing is the health:

With the delegate created I can then make an event based around it, so here I made an event called healthChanged:

Now I need to trigger this event, and I want to trigger the event from a function called onHealthChanged, we need this function because I need to check if something is listening to the event before it is triggered:
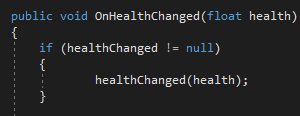
Then in the enemy script I added a public override called TakeDamage, so now the enemy is overriding takeDamage and calls the base damage:
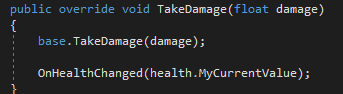
Next in the UI manager I created something called updateTargetFrame which will update the health on the target frame:
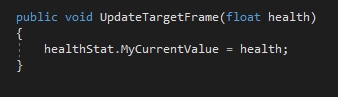
This next line of code allows the game to listen to the event, which in turn activates the function and the health will update as it should with new health values:

Here is what the unit frame looks like in my game currently, as you can see when I select the target GUI pops up in the center middle of the game screen which displays the icon of the target and also its health, and as you can also see the health updates as it should both above the enemy and within the enemy target frame:

Script
First things first I needed to create a game object for the GUI which was done in the UIManager script.

Then I created a new function called showTargetFrame which will show the enemies target frame once clicking on the target. This function works for not only enemy targets but friendly targets such as quest givers or vendors:

This line of code sets the frame to show:

Next we need to call this function, this can be done in the gameManager script inside the click target function. First thing I did was create a private static instance in UIManager called UIManager:

Next I made it so if instance is null then we have to set it. This makes sure when I access the UIManager through myInstance we check if the instance is set if not we set it then return it:
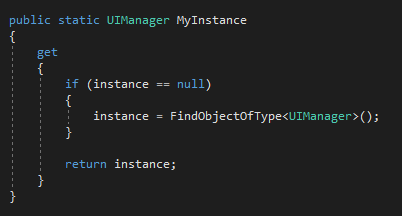
Then within gameManager I put this line of code which allows the gameManager script with the click function to access my UIManager script and the showTarget functionality:

After this the target GUI will display at the top of the screen when selecting it. Now I need to make it so when we unselect the target the GUI goes away. Pretty simple way of doing that:
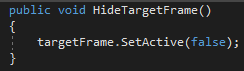
Currently the health doesn't update, so I will have to initialize the health on the target frame. To do this I will create a health stat in the character script:
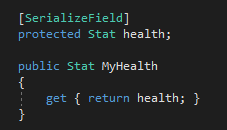
Then within the show target frame function I create this line of code which initializes the health for the selected target which sets its max health value and its current value:

Then to allow this functionality to work from the get go I added this line of code within the void start within the ui manager script:

Now when I go into the game and select the target the correct information about the target will be displayed at the top. However at this stage there is no functionality for updating the health once the player damages it, so I will need to add that.
So to do this I will be creating a delegate, so in the NPC script I created a delegate called HealthChanged, and the function that it will be changing is the health:

With the delegate created I can then make an event based around it, so here I made an event called healthChanged:

Now I need to trigger this event, and I want to trigger the event from a function called onHealthChanged, we need this function because I need to check if something is listening to the event before it is triggered:
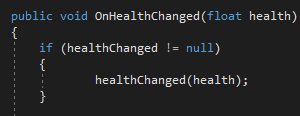
Then in the enemy script I added a public override called TakeDamage, so now the enemy is overriding takeDamage and calls the base damage:
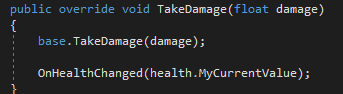
Next in the UI manager I created something called updateTargetFrame which will update the health on the target frame:
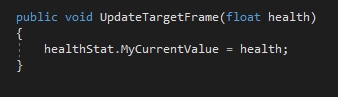
This next line of code allows the game to listen to the event, which in turn activates the function and the health will update as it should with new health values:

Here is what the unit frame looks like in my game currently, as you can see when I select the target GUI pops up in the center middle of the game screen which displays the icon of the target and also its health, and as you can also see the health updates as it should both above the enemy and within the enemy target frame:

Comments
Post a Comment