How will I create my map?
The map is going to based on images and on the images each of the pixels will represent one tile in the game world, so if the map was 100x100 then I would need to create an image that is 100x100 pixels, this can be done in many different graphic softwares but I personally will be using photoshop as I know how to use it relatively well.
Here is an example of what it looks like in Photoshop:
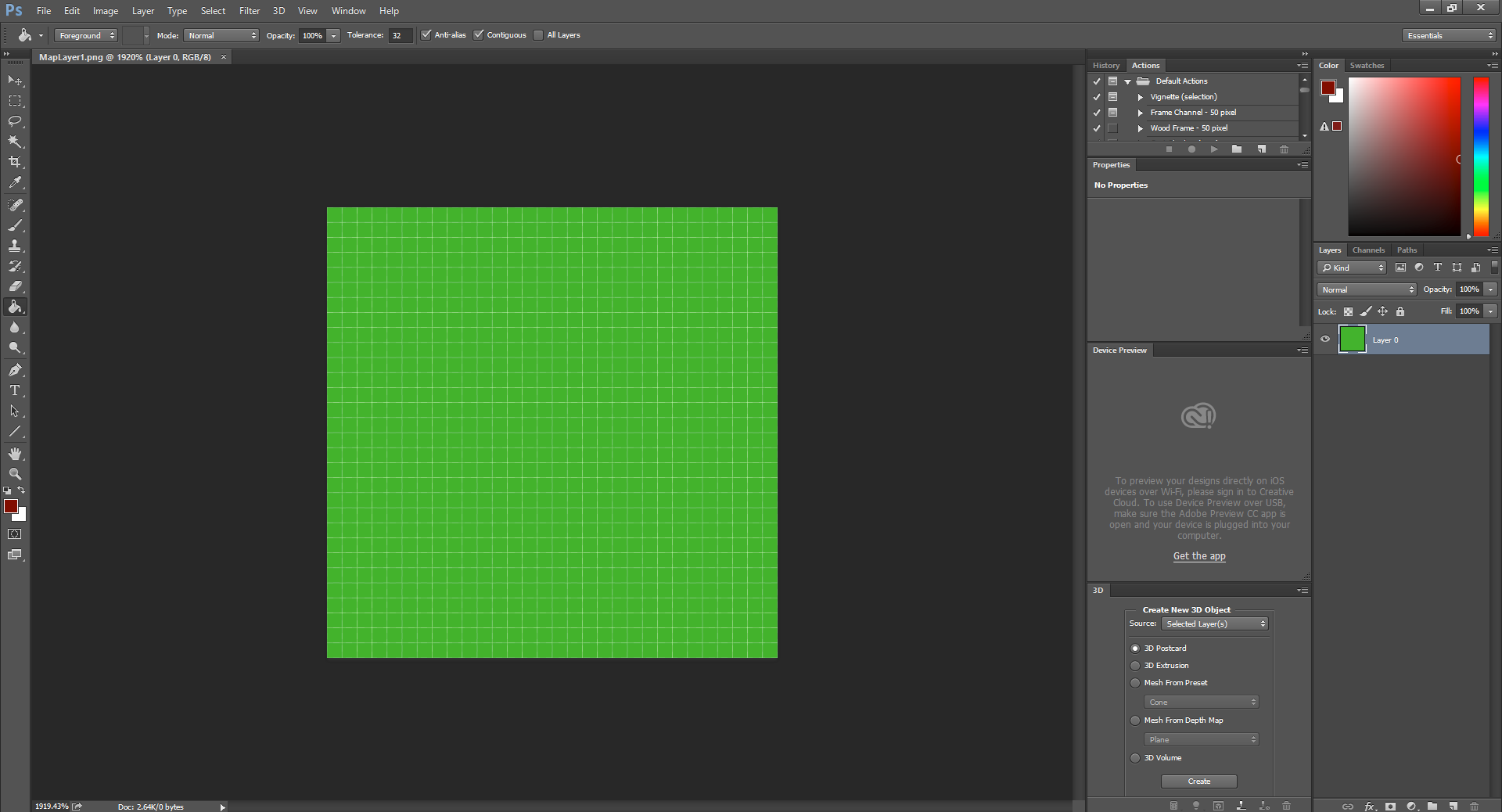
Editing the map is super easy in Photoshop and every change I make to this map will change the asset in Unity also, so if I add a path using the pencil tool and save it, it will show up in my game also:
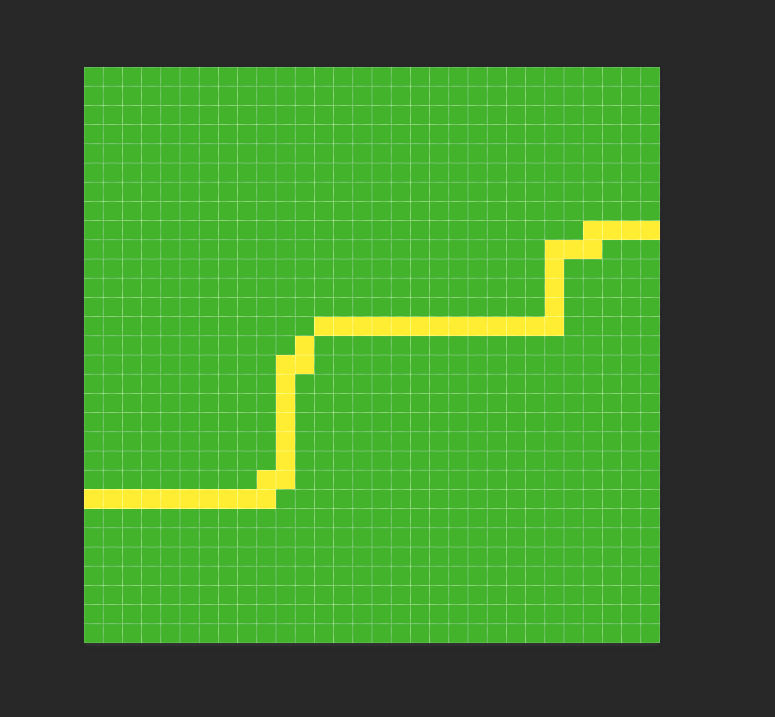
Or, if I wanted to create water in my world, that can easily be done also using the same technique with a different color (blue):
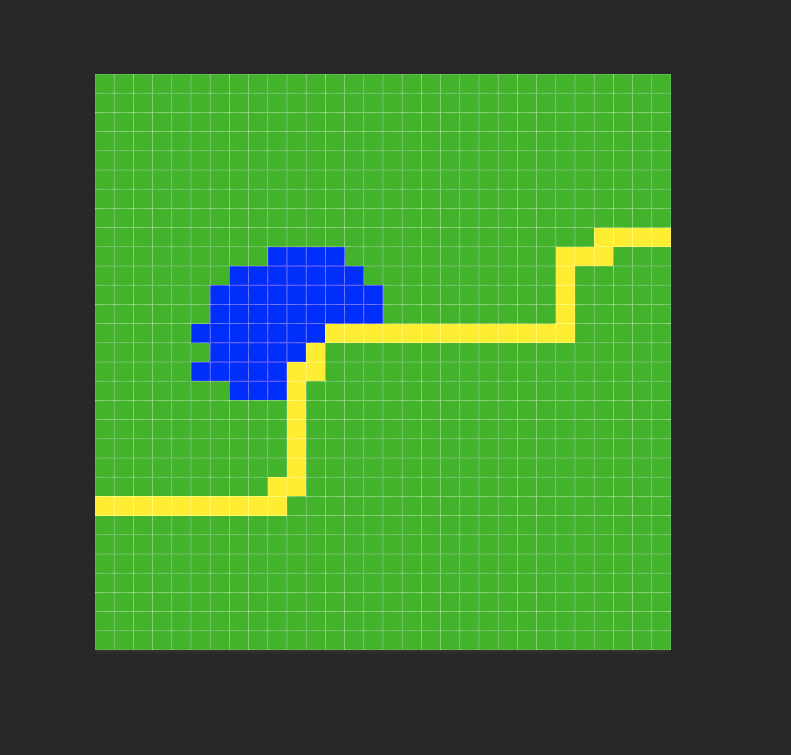
So for all this to look nice I will need some map sprites to go with all the colors I have previously shown. Here I have added a grass tile sprite in a new folder I created where I will put all of my world map sprites:
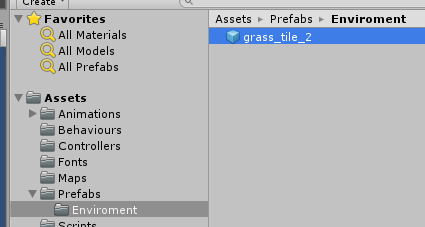
Code
I needed to create a new script which will be a manager that will generator the maps for me, I named this script level manager:
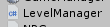
The levels are going to based around a grid of pixels, so because of this I need to create a private Texture2D and an array called mapData:

The reason for the array is because I would like to generator more layers, so the grass and such is the tiles and on top of the tiles will be placed things such as the character, skeletons, trees etc.
Now for the map, the image I shown earlier in Photoshop is important because we will be using the green color used in Photoshop and code it in such way that the green color will generate into grass tiles:
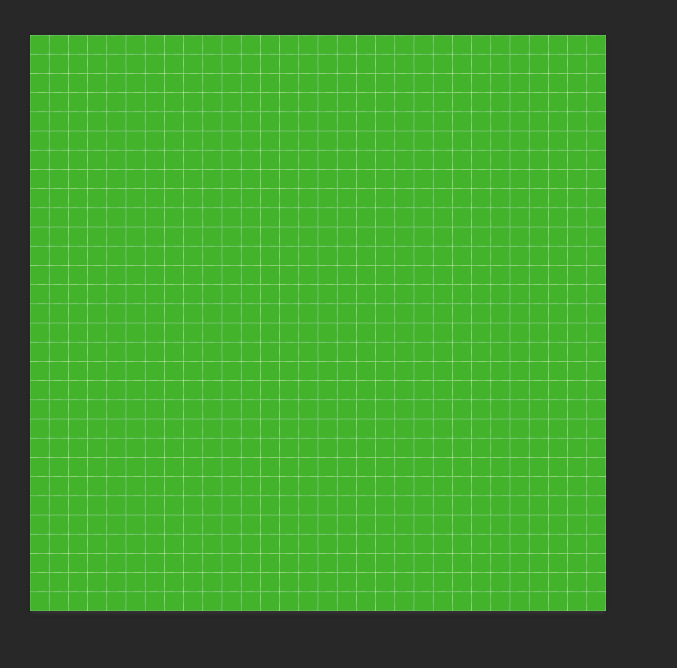
This image was saved into the maps folder of the assets as 'MapLayer1.png':
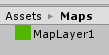
Next I have to change the settings of the texture type, because Unity is going to have to read all the data on the texture and get the pixels out and based on the pixels it needs to place a tile within the game. The two things I changed are texture type to default, and read/write enabled:
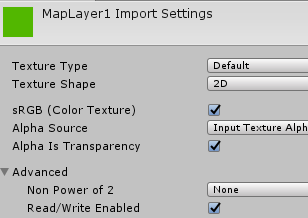
Next I created another class called mapElement, this is a tile, it can be water, grass stone etc. that will be placed in our world:

Then I needed to create a tileTag which is used to recognize what type of tile we are trying to set:
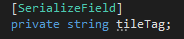
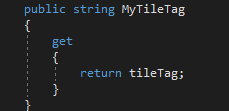
Then I created a color which is used for placing the tile, in Photoshop I explained that different colors are used for different tiles, Unity will look at each pixel and return the color for that pixel, based on the color a tile is placed e.g green for grass:
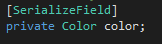
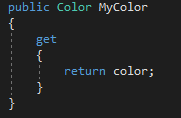
Then I created the prefab that I use to spawn the tile in the world, so Unity will read the color then use the prefab to place it in the game world:

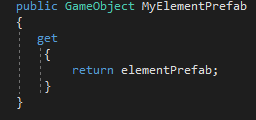
And then next I need defaultSprite because the default sprite is the ruler for the size of all tiles for the game and it will measure how much space needs to be between our tiles:
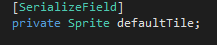
I also need the starting point in my world before I can place anything, and i would like to place this based off the camera. So I created a vector3 start position and in it will return the camera main screen to world point at 0,0 (which is the bottom left of the camera) so I will always see the tile map when I start the game:
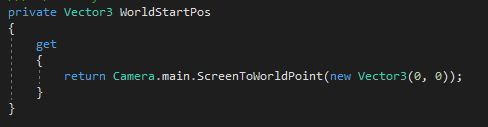
Next I added the level manager within Unity into the game world so it can function:
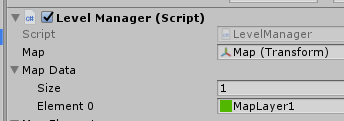
Next I added the grass title sprite to the default tile as that is going to be the first layer tile, I then chose the color of the world map layer to the color of what I created in photoshop so it can then spawn the grass tiles on that specific color (I did this by grabbing the hex color from Photoshop and pasting into Unity:
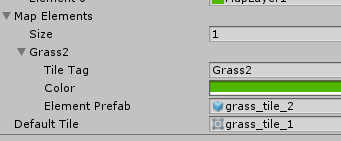
I then created a new function called generateMap, this will generate the map for me. Within this function i created a forLoop for the map data which checks through all the layers of the map as I will be having more than 1 later. The map has x values and y values which go up and across, so I created a for loop which checks through all the coordinates of the different pixels on the layer on x and y. Next it needs to grab the color it is currently looking at, so for this is used mapData getPixel x and y. The map newElement checks if I have a tile that suits the color of the pixel on the map using the prefab, so if the color green is on the map, that will be suited to the grass tile prefab. And then if newElement doesn't equal null then spawn the tile, it then calculates the position of the x and y of the tile, creates the tile, sets the tiles position and the finally make the tile a child of the map.
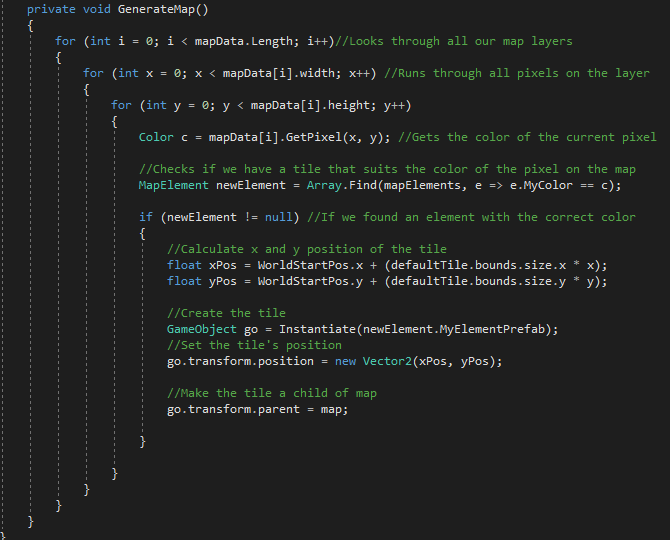
So now that the algorithm is created it will now spawn in a tile map for me based on the colors I have chosen for the world map! I can now add different things like water, trees and pathways all using different colors and assets. Here is what my game world currently looks like with just one tile asset used:

The map is going to based on images and on the images each of the pixels will represent one tile in the game world, so if the map was 100x100 then I would need to create an image that is 100x100 pixels, this can be done in many different graphic softwares but I personally will be using photoshop as I know how to use it relatively well.
Here is an example of what it looks like in Photoshop:
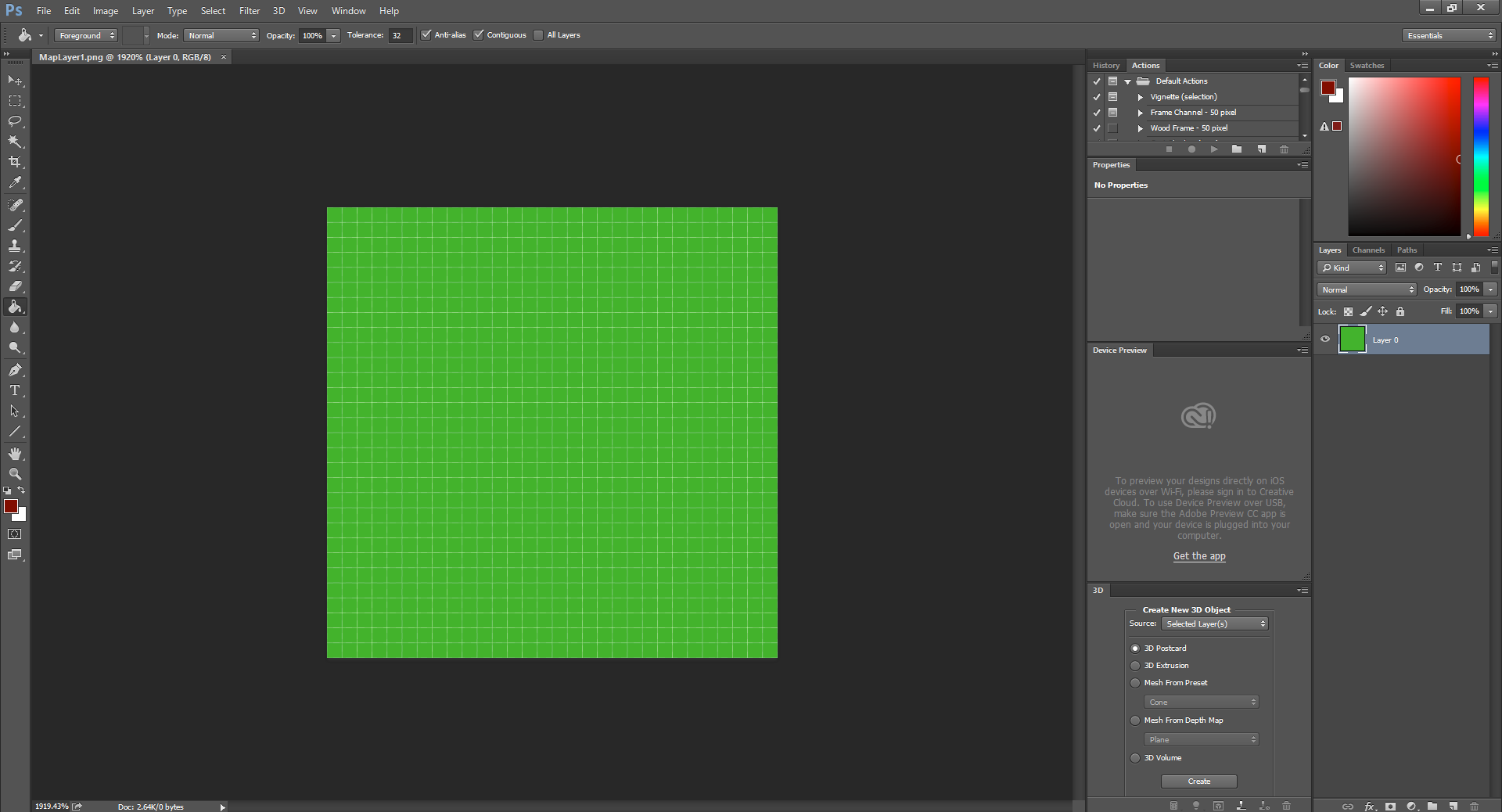
Editing the map is super easy in Photoshop and every change I make to this map will change the asset in Unity also, so if I add a path using the pencil tool and save it, it will show up in my game also:
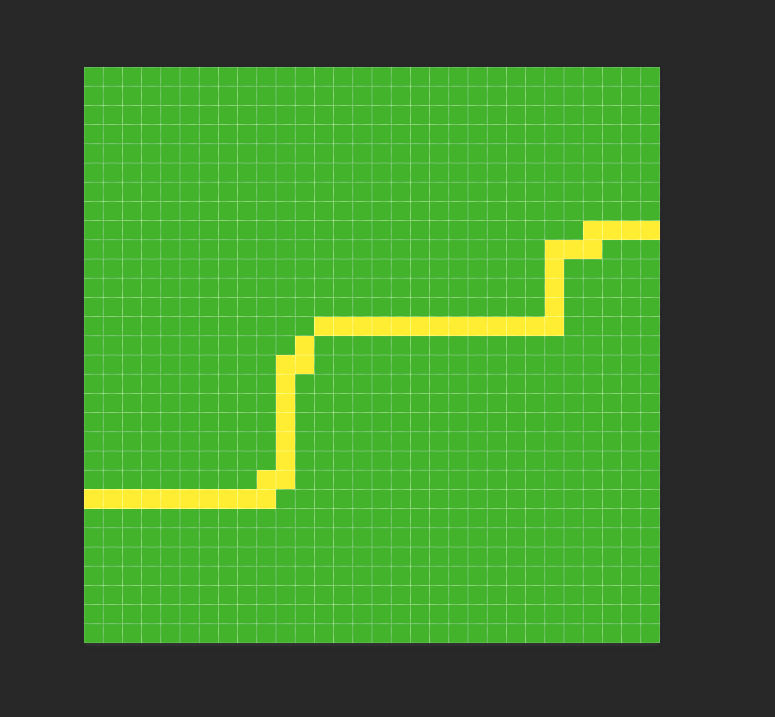
Or, if I wanted to create water in my world, that can easily be done also using the same technique with a different color (blue):
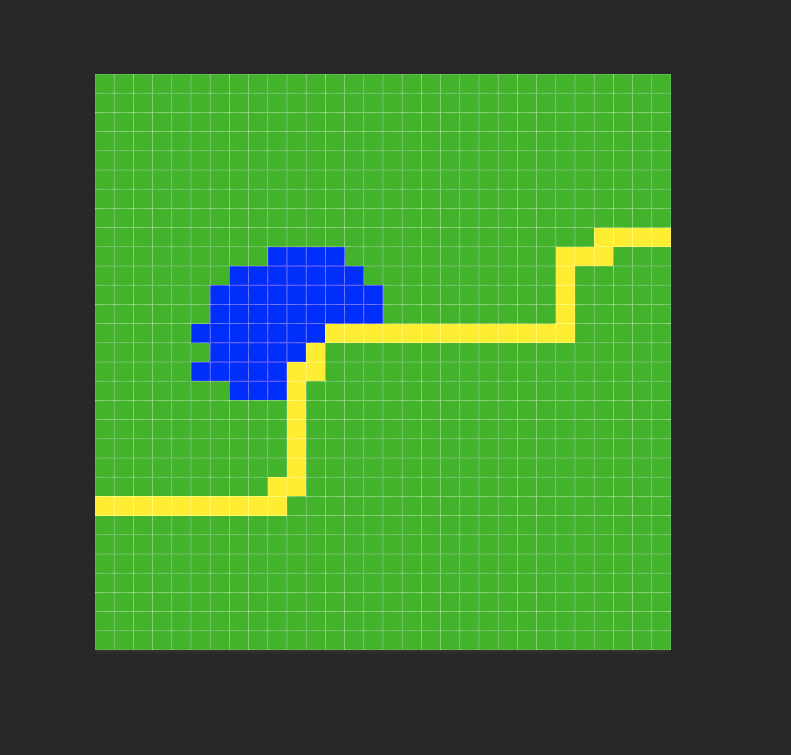
So for all this to look nice I will need some map sprites to go with all the colors I have previously shown. Here I have added a grass tile sprite in a new folder I created where I will put all of my world map sprites:
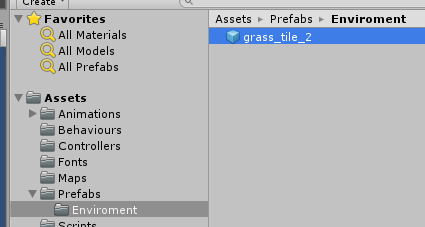
Code
I needed to create a new script which will be a manager that will generator the maps for me, I named this script level manager:
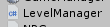
The levels are going to based around a grid of pixels, so because of this I need to create a private Texture2D and an array called mapData:

The reason for the array is because I would like to generator more layers, so the grass and such is the tiles and on top of the tiles will be placed things such as the character, skeletons, trees etc.
Now for the map, the image I shown earlier in Photoshop is important because we will be using the green color used in Photoshop and code it in such way that the green color will generate into grass tiles:
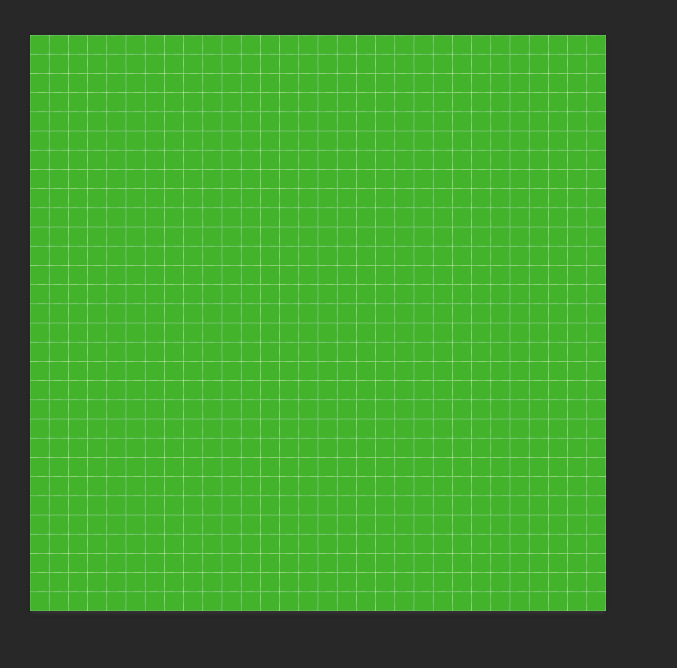
This image was saved into the maps folder of the assets as 'MapLayer1.png':
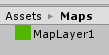
Next I have to change the settings of the texture type, because Unity is going to have to read all the data on the texture and get the pixels out and based on the pixels it needs to place a tile within the game. The two things I changed are texture type to default, and read/write enabled:
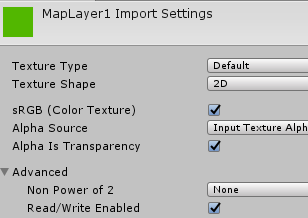
Next I created another class called mapElement, this is a tile, it can be water, grass stone etc. that will be placed in our world:

Then I needed to create a tileTag which is used to recognize what type of tile we are trying to set:
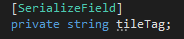
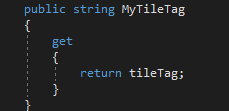
Then I created a color which is used for placing the tile, in Photoshop I explained that different colors are used for different tiles, Unity will look at each pixel and return the color for that pixel, based on the color a tile is placed e.g green for grass:
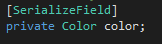
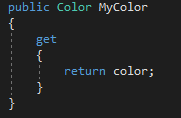
Then I created the prefab that I use to spawn the tile in the world, so Unity will read the color then use the prefab to place it in the game world:

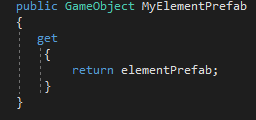
And then next I need defaultSprite because the default sprite is the ruler for the size of all tiles for the game and it will measure how much space needs to be between our tiles:
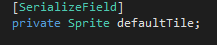
I also need the starting point in my world before I can place anything, and i would like to place this based off the camera. So I created a vector3 start position and in it will return the camera main screen to world point at 0,0 (which is the bottom left of the camera) so I will always see the tile map when I start the game:
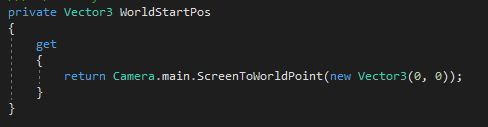
Next I added the level manager within Unity into the game world so it can function:
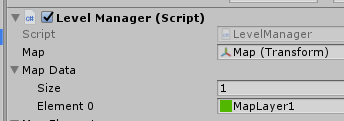
Next I added the grass title sprite to the default tile as that is going to be the first layer tile, I then chose the color of the world map layer to the color of what I created in photoshop so it can then spawn the grass tiles on that specific color (I did this by grabbing the hex color from Photoshop and pasting into Unity:
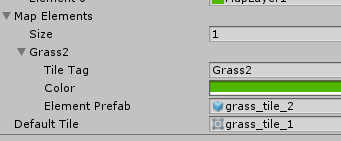
I then created a new function called generateMap, this will generate the map for me. Within this function i created a forLoop for the map data which checks through all the layers of the map as I will be having more than 1 later. The map has x values and y values which go up and across, so I created a for loop which checks through all the coordinates of the different pixels on the layer on x and y. Next it needs to grab the color it is currently looking at, so for this is used mapData getPixel x and y. The map newElement checks if I have a tile that suits the color of the pixel on the map using the prefab, so if the color green is on the map, that will be suited to the grass tile prefab. And then if newElement doesn't equal null then spawn the tile, it then calculates the position of the x and y of the tile, creates the tile, sets the tiles position and the finally make the tile a child of the map.
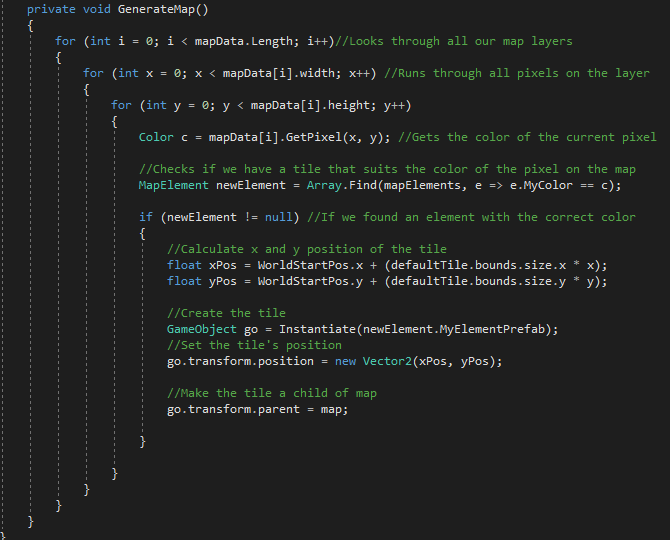
So now that the algorithm is created it will now spawn in a tile map for me based on the colors I have chosen for the world map! I can now add different things like water, trees and pathways all using different colors and assets. Here is what my game world currently looks like with just one tile asset used:

Comments
Post a Comment